As a developer, encountering errors while coding is inevitable. One common error that C programmers come across is the "assignment makes integer from pointer without a cast" error. This error message can be frustrating and time-consuming to resolve, but with the right tips and solutions, it can be easily fixed.
Understanding the Error Message
Before we dive into the tips and solutions for fixing this error, let's first understand what it means. The "assignment makes integer from pointer without a cast" error occurs when a pointer is assigned to an integer without a proper type cast. This error message is often accompanied by a warning message that looks like this:
warning: assignment makes integer from pointer without a cast
This warning message is telling the programmer that the code is trying to assign a pointer value to an integer variable without casting the pointer to the correct type.
Tips for Fixing the Error
Here are some tips to help you fix the "assignment makes integer from pointer without a cast" error:
Tip #1: Check Your Pointer Types
Make sure that the pointer you are trying to assign to an integer variable is of the correct data type. If the pointer is pointing to a different data type, you will need to cast it to the correct type before assigning it to the integer variable.
Tip #2: Use the Correct Syntax
When casting a pointer to a different data type, make sure to use the correct syntax. The syntax for casting a pointer to an integer is (int) pointer
.
Tip #3: Use the Correct Assignment Operator
Make sure that you are using the correct assignment operator. The assignment operator for pointers is =
while the assignment operator for integers is ==
.
Tip #4: Check Your Code for Errors
Double-check your code for errors. Sometimes, the "assignment makes integer from pointer without a cast" error can be caused by a syntax error or a missing semicolon.
Solutions for Fixing the Error
Now that you have some tips for fixing the "assignment makes integer from pointer without a cast" error, let's look at some solutions.
Solution #1: Cast the Pointer to the Correct Type
To fix this error, you need to cast the pointer to the correct type before assigning it to the integer variable. Here's an example:
int *ptr;
int num;
ptr = (int*) malloc(sizeof(int));
*num = (int) ptr;
In this example, the pointer is cast to an integer using the (int)
syntax before it is assigned to the num
variable.
Solution #2: Declare the Integer Variable as a Pointer
Another solution is to declare the integer variable as a pointer. Here's an example:
int *num;
int *ptr;
ptr = (int*) malloc(sizeof(int));
num = ptr;
In this example, the num
variable is declared as a pointer, and the ptr
variable is assigned to it without casting.
FAQ
Q1: What causes the "assignment makes integer from pointer without a cast" error?
A: This error occurs when a pointer is assigned to an integer variable without being cast to the correct data type.
Q2: How do I cast a pointer to an integer in C?
A: To cast a pointer to an integer in C, use the (int)
syntax.
Q3: Why is my code still giving me the same error message even after I cast the pointer to the correct type?
A: Double-check your code for syntax errors and missing semicolons. Sometimes, these errors can cause the same error message to appear even after you have cast the pointer to the correct type.
Q4: Can I declare the integer variable as a pointer to fix this error?
A: Yes, you can declare the integer variable as a pointer to fix this error.
Q5: What is the correct assignment operator for pointers and integers in C?
A: The assignment operator for pointers is =
while the assignment operator for integers is ==
.
Conclusion
The "assignment makes integer from pointer without a cast" error can be frustrating, but with the right tips and solutions, it can be easily fixed. By understanding the error message and following the tips and solutions provided in this guide, you can resolve this error and improve the functionality of your code.
Related Links
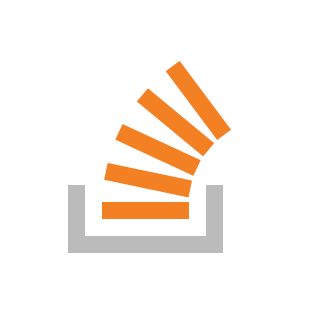