There are several ways to fix a "shuffle deck of cards" error in C++, but a common approach is to use the built-in random number generator and the "swap" function to shuffle the elements of an array or vector that represents the deck of cards. Here is an example of how you might shuffle a deck of cards represented by an array of integers:
#include <iostream>
#include <algorithm>
#include <random>
const int NUM_CARDS = 52;
int deck[NUM_CARDS];
int main() {
// Initialize the deck of cards
for (int i = 0; i < NUM_CARDS; i++) {
deck[i] = i;
}
// Shuffle the deck using the Fisher-Yates algorithm
std::random_device rd;
std::mt19937 g(rd());
for (int i = NUM_CARDS - 1; i > 0; i--) {
std::uniform_int_distribution<int> dist(0, i);
int j = dist(g);
std::swap(deck[i], deck[j]);
}
// Print the shuffled deck
for (int i = 0; i < NUM_CARDS; i++) {
std::cout << deck[i] << " ";
}
return 0;
}
This code uses the Fisher-Yates algorithm to shuffle the deck of cards. The basic idea of the algorithm is to iterate through the deck of cards starting from the last card, and for each card, randomly swap it with one of the cards before it. The random_device
and mt19937
classes are used to generate random numbers, and the uniform_int_distribution
class is used to generate a random index between 0 and the current index of the card being shuffled. Finally, the swap
function is used to swap the current card with the randomly selected card.
Here, we are using mt19937
generator and uniform_int_distribution
as our random generator. The function mt19937
is a random number generator with a Mersenne Twister algorithm that has a large period and good statistical properties. uniform_int_distribution
is used to get uniformly distributed random number in the range specified. And the function swap
is used to swap the position of two cards in the deck array.
It's worth noting that this code will shuffle the deck in-place, meaning that the original array will be modified. If you need to keep the original deck intact, you should make a copy of the deck before shuffling it.
Related Issue:
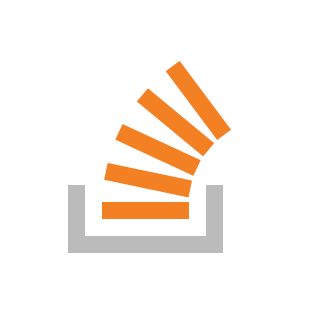
Frequently Asked Questions About C++ Shuffle Deck Of Cards
- How do I shuffle a deck of cards in C++?
There are several ways to shuffle a deck of cards in C++, including using the built-in random number generator and the "swap" function, or using the shuffle
function from the algorithm
library. Both methods involve iterating through the deck of cards and swapping the elements in a random order.
2. Can I use the random_shuffle
function to shuffle a deck of cards in C++?
The random_shuffle
function is deprecated in C++11 and removed in C++17, and it is not recommended to use it as it produces non-random shuffle in some cases.
3. Can I shuffle a deck of cards without using a random number generator?
It is not possible to shuffle a deck of cards without using a random number generator or some other randomizing mechanism.
4. How do I ensure that the shuffle is truly random?
To ensure that the shuffle is truly random, you can use a cryptographically secure random number generator such as random_device
class in C++. This class uses a hardware random number generator to seed the random number generator.
5. How do I shuffle a deck of cards represented by an object?
You can use the same methods to shuffle a deck of cards represented by an object as you would for an array or vector, but you will need to create a suitable container to hold the objects, such as a vector or list, and use the appropriate access methods to swap the objects.