The "dangling meta character '*' near index" error is typically caused by using the * character in a regular expression without escaping it properly. The * character is a special character in regular expressions that is used to match zero or more occurrences of the preceding character. If it is not escaped, it will cause an error.
To fix this error, you need to escape the * character by placing a backslash () in front of it. For example, if your regular expression is currently ".*", you would need to change it to ".*" to properly escape the * character.
Alternatively, you can use the re.escape()
method to escape all the special characters in a string that you want to use as a regular expression.
Here is an example of how to use re.escape()
:
import re
string_to_escape = ".*+?"
escaped_string = re.escape(string_to_escape)
print(escaped_string)
# Output: "\\.\*\+\?"
Also, you can use []
to group the special characters that you want to use as a regular expression.
Here is an example:
import re
string_to_escape = ".*+?"
pattern = "[" + string_to_escape + "]"
print(pattern)
# Output: "[.*+?]"
This will create a regex pattern that will match any of the characters contained within the square brackets, which will include * and +, but will not treat them as special characters.
After you have properly escaped the * character, you can then use the regular expression in your code without encountering the "dangling meta character '*' near index" error.
It is important to note that when working with regular expressions, it is always a good practice to test your regular expressions using a tool such as a regex tester before using them in your code. This will help you to identify and fix any errors in your regular expressions before they cause problems in your code.
Another thing to keep in mind is the context where you are using the regular expression, if you are using the regex pattern in the re.search()
or re.match()
method, the pattern should match the entire string, otherwise you may get unexpected results or errors.
Lastly, when you are done with your regex pattern, make sure to compile it, for better performance and to make it easier to use multiple times.
import re
pattern = re.compile(".*")
By following these steps, you can fix the "dangling meta character '*' near index" error and use regular expressions in your code without encountering any issues.
Related Issue Link:
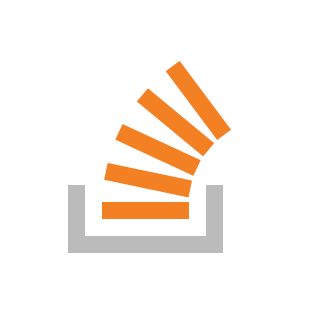
Frequently Asked Questions About Dangling Meta Character Error
- What causes the "dangling meta character '*' near index" error?
This error is typically caused by using the * character in a regular expression without escaping it properly. The * character is a special character in regular expressions that is used to match zero or more occurrences of the preceding character. If it is not escaped, it will cause an error.
2. How can I fix the "dangling meta character '*' near index" error?
To fix this error, you need to escape the * character by placing a backslash () in front of it. Alternatively, you can use the re.escape()
method to escape all the special characters in a string that you want to use as a regular expression. Also, you can use []
to group the special characters that you want to use as a regular expression.
3. Is there any tool to test regular expressions before using them in code?
Yes, there are many regex testing tools available online that you can use to test your regular expressions before using them in your code. These tools will help you to identify and fix any errors in your regular expressions before they cause problems in your code.
4. Should I compile the regular expression pattern after using it?
Yes, it is a good practice to compile the regular expression pattern after using it for better performance and to make it easier to use multiple times.
5. Can the error also occur when I haven't included anything for the regular expression to match?
Yes, the error can also occur when you're using a regular expression to match a string, but you haven't included anything for the regular expression to match. The * character is a special character in regular expressions that is used to match zero or more occurrences of the preceding character, so if there is no preceding character, the error may occur.