This comprehensive guide will walk you through the process of resolving the "Expected declaration before '}' token" error in your code. This error is commonly encountered when working with C, C++, or JavaScript languages, and occurs when the compiler encounters an unexpected closing brace that does not correspond to an opening brace within the same scope.
Table of Contents
Understanding the Error
The "Expected declaration before '}' token" error occurs when there is a mismatch between the opening and closing braces in your code. This could be due to a missing opening brace, an extra closing brace, or a misplaced closing brace. In some cases, it might also be caused by incorrect nesting of braces.
To fix this error, you need to identify the cause and ensure that all opening and closing braces are correctly paired and nested.
Identifying the Cause
Missing Opening Brace: If you forget to include an opening brace for a code block, the compiler will treat the closing brace as unexpected and throw an error.
int main() {
// Code here...
// Missing opening brace
}
Extra Closing Brace: If you accidentally include an extra closing brace in your code, the compiler will not be able to find a matching opening brace and will throw an error.
int main() {
// Code here...
}
} // Extra closing brace
Misplaced Closing Brace: If a closing brace is placed in the wrong position, the compiler will not be able to match it with the corresponding opening brace and will throw an error.
```c
int main() {
// Code here...
} // Misplaced closing brace
}
```
Step-by-Step Solutions
Follow these steps to resolve the "Expected declaration before '}' token" error in your code:
Carefully review your code, paying close attention to the opening and closing braces. Look for any missing, extra, or misplaced braces.
Ensure that all opening braces have a corresponding closing brace, and that they are correctly nested. Use proper indentation to make this task easier.
If you find a missing opening brace, add it in the appropriate position.
If you find an extra closing brace, remove it.
If you find a misplaced closing brace, move it to the correct position.
Once you have made the necessary changes, recompile your code and ensure that the error has been resolved.
FAQs
1. How do I find the line number where the error occurred?
Most compilers will display the line number where the error was detected in the error message. You can use this information to quickly locate the problematic section of your code.
2. How can I prevent this error from happening in the future?
To prevent this error, always make sure to properly pair and nest your opening and closing braces. Additionally, using a good IDE or text editor with syntax highlighting and automatic indentation can help you quickly identify any brace-related issues.
3. How do I fix this error in an IDE like Visual Studio or Eclipse?
IDEs like Visual Studio or Eclipse typically provide error detection and code analysis tools that can help you identify and fix brace-related issues. These tools may automatically highlight problematic lines, or provide suggestions for resolving the issue.
4. Can this error occur in languages other than C, C++, or JavaScript?
Yes, this error can occur in any programming language that uses braces to define code blocks, such as Java or Swift. The steps to resolve the error are similar across all languages.
5. Is there a difference between braces, curly brackets, and curly braces?
No, these terms all refer to the same punctuation marks: {
and }
. They are used interchangeably to describe the opening and closing braces used in programming languages to define code blocks.
Related Links
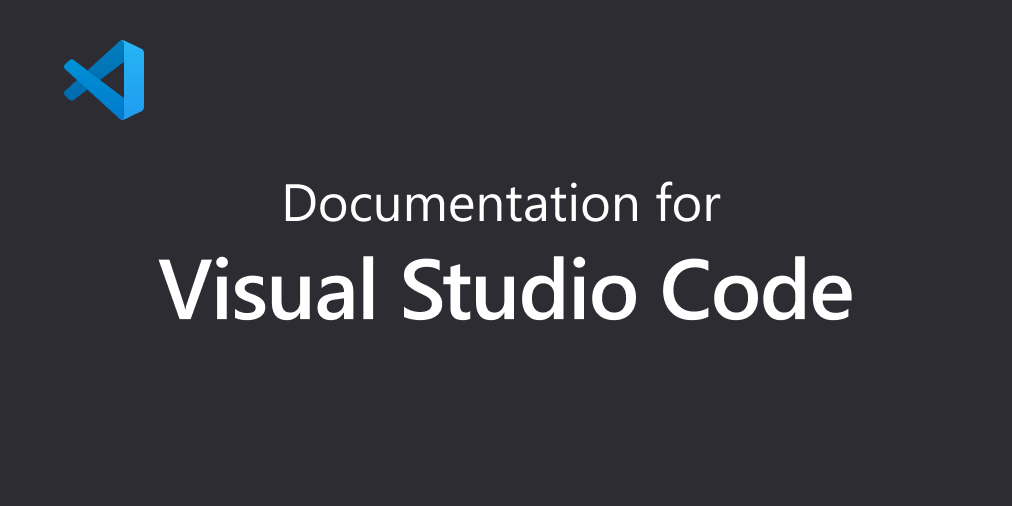