Introduction
C++ pointers are an advanced concept that can often be difficult to understand. By copying a character to a pointer, we are able to assign a value to the pointer, which can then be used by our program. Learning how to do this correctly will ensure that your program is correctly allocating data and that it runs without any errors.
What Are Pointers?
In C++, pointers are objects that store the address of another variable. They are commonly used to store the address of an array. All pointers have a single data type, and the value stored in the pointer can be accessed by using the pointer's variable name.
Step-by-Step Guide
- Create a new character variable by using the char keyword followed by the variable name:
char myChar;
- Assign a value to the char variable and use the asterisk operator to initialize a pointer to the character:
myChar = 'a'; char *charPtr = &myChar;
- Use the pointer to copy the char value to another memory location:
*charPtr = myChar;
FAQ
What is the syntax for initializing a char pointer?
The syntax for initializing a char pointer is char *pointerName = &variableName
, where pointerName
is the name of the pointer and variableName
is the name of the char variable you wish to store in the address of the pointer.
How do I assign a value to a char pointer?
Your char pointer will contain the value of the char variable that you have assigned to it. Therefore, you can assign a value to the char pointer by assigning a value to the char variable. For example, if you have a char variable called myChar
and you wish to assign a value a
to the char pointer charPtr
, you would use the following syntax:myChar = 'a'; char *charPtr = &myChar;
How do I copy a character to a pointer?
To copy a character to a pointer, you must use the asterisk operator to assign the char variable's value to the pointer. For example, to assign the value of myChar
to the pointer charPtr
, you would use the following syntax:*charPtr = myChar;
What is the importance of creating a char pointer?
Creating a char pointer enables you to access the value of the char variable without having to explicitly use the variable name. This allows for greater flexibility and easier programming.
What is the difference between a char and a pointer?
A char is a data type that stores a single character, and a pointer is an object that stores the address of another variable. A char pointer is a pointer that holds the address of a char variable.
Related Links
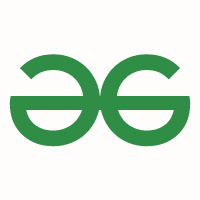