The "fakepath" issue when uploading files is caused by a security feature in web browsers that prevents the full path of a client's file from being exposed. Instead of showing the full path, the browser will display "fakepath" in the file input field.
To remove the "fakepath" and show the actual file name, you can use the JavaScript .value
property of the file input element.
Here is an example of how to do this in JavaScript:
// Get the file input element
var fileInput = document.getElementById("fileInput");
// Get the value of the file input (i.e. the file name)
var fileName = fileInput.value;
// Use the file name to update a label or other element
document.getElementById("fileNameLabel").innerHTML = fileName;
You can also use the FileReader
API to read the file and display its name in the page.
Here is an example:
var input = document.getElementById("fileInput");
var file = input.files[0];
var fr = new FileReader();
fr.onload = function () {
var fileName = file.name;
document.getElementById("fileNameLabel").innerHTML = fileName;
};
fr.readAsDataURL(file);
Please note that this only works for the client-side and will not affect the actual file name on the server.
Please be aware that the above code just show the file name on the page, to use this file name on server side you need to send this file name along with the file to the server and use that file name on server side.
In order to use the file name on the server side, you will need to include it as part of the form data that is sent to the server when the file is uploaded. This can be done using the FormData
object in JavaScript.
Here is an example of how to do this:
// Get the file input element
var fileInput = document.getElementById("fileInput");
// Create a new FormData object
var formData = new FormData();
// Add the file to the FormData object
formData.append("file", fileInput.files[0]);
// Add the file name to the FormData object
formData.append("fileName", fileInput.value);
// Use the FormData object to send the file and file name to the server
var xhr = new XMLHttpRequest();
xhr.open("POST", "upload.php", true);
xhr.send(formData);
On the server side, you can access the file and file name using the $_FILES
and $_POST
arrays in PHP.
Here is an example of how to do this in PHP:
$file = $_FILES["file"];
$fileName = $_POST["fileName"];
// Use the file and file name to save the file to the server
move_uploaded_file($file["tmp_name"], "uploads/" . $fileName);
This is a basic example, but it should give you an idea of how to include the file name as part of the form data when uploading a file, and how to access the file name on the server side.
Please note that you should validate the file name and file type on server side before saving the file to the server, to prevent any security issues.
Related Issue:
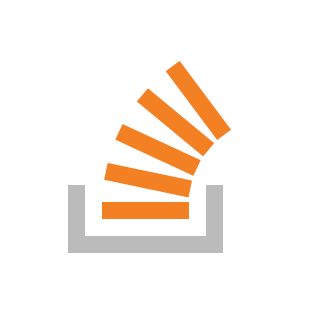
Frequently Asked Questions About How To Remove Fakepath In File Upload
What causes the "fakepath" issue when uploading files?
The "fakepath" issue is caused by a security feature in web browsers that prevents the full path of a client's file from being exposed. Instead of showing the full path, the browser will display "fakepath" in the file input field.
How do I remove the "fakepath" and show the actual file name?
You can use the JavaScript .value
property of the file input element or the FileReader
API to read the file and display its name in the page. Additionally you have to send this file name along with the file to the server.
How can I use the file name on the server side?
You will need to include the file name as part of the form data that is sent to the server when the file is uploaded. This can be done using the FormData
object in JavaScript and on the server side you can access the file name using the $_FILES
and $_POST
arrays in PHP.
Is it safe to use the file name on the server side?
It is important to validate the file name and file type on the server side before saving the file to the server to prevent any security issues.
How do I handle multiple files with different names?
You can use a loop to iterate through all the files and append them to the FormData
object along with their respective file names. On the server side, you can use a loop to iterate through the $_FILES
array and process each file individually.
Can I use this method for older browsers?
The method I described uses JavaScript and the FormData
object, which are supported by most modern browsers, but may not be supported by older browsers. If you need to support older browsers, you may need to use a different method, such as a traditional form submission with a hidden input field for the file name.
Is it possible to remove the "fakepath" issue in the server-side?
The "fakepath" issue is caused by a security feature in web browsers and cannot be removed on the server-side. The only way to remove it is by using client-side methods like the ones I described earlier.
Can I use this method for mobile devices?
The method I described should work on mobile devices as well as desktop browsers, as long as the device has a modern browser that supports the FormData
object and JavaScript.
Is there any other best practice to handle file uploads?
Along with the method I described, there are other best practices you should follow when handling file uploads, such as validating the file type and size, and ensuring that the uploaded files are stored in a secure location. Additionally, you may want to consider using a library or framework that provides additional security and functionality when handling file uploads.
Can you give an example of how to handle the file uploads using a framework?
Sure, here's an example of how to handle file uploads using the Flask framework in Python:
from flask import Flask, request
app = Flask(__name__)
@app.route('/upload', methods=['POST'])
def upload():
file = request.files['file']
file.save('uploads/' + file.filename)
return 'File uploaded successfully'
In this example, the file is being uploaded via a POST request to the /upload
endpoint. The file is then being saved to the 'uploads/' directory with its original file name.
Please note that these are just examples and you should always consult the documentation of the specific framework or library you are using for the best practices and implementation details.