As a C++ programmer, you may have encountered the error message "invalid conversion from 'char*' to 'char'" while writing your code. This error can be frustrating, especially if you don't know how to fix it. In this guide, we'll provide you with easy solutions to this error.
What Causes the "Invalid Conversion from Char* to Char" Error?
This error occurs when you try to assign a string literal (a char*) to a character variable (a char). In C++, a string literal is represented by a pointer to an array of characters, while a character variable is a single character. Therefore, you can't assign a string literal to a character variable directly.
Solution 1: Use Single Quotes Instead of Double Quotes
One solution to this error is to use single quotes (') instead of double quotes (") when declaring character variables. Single quotes represent a single character, while double quotes represent a string literal. Here's an example:
char myChar = 'a'; // Correct
char myChar = "a"; // Incorrect
Solution 2: Use the Index Operator to Access a Single Character
Another solution is to use the index operator to access a single character in the string literal. Here's an example:
char* myString = "Hello";
char myChar = myString[0]; // Gets the first character of the string
Solution 3: Use the std::string Class
Finally, you can use the std::string class to store and manipulate strings in C++. The std::string class provides a convenient way to handle strings, and you can easily convert a std::string to a char* if needed. Here's an example:
std::string myString = "Hello";
char myChar = myString[0]; // Gets the first character of the string
const char* myCharPtr = myString.c_str(); // Converts the string to a char*
FAQ
Q1: What is a string literal?
A string literal is a sequence of characters enclosed in double quotes (""). In C++, a string literal is represented by a pointer to an array of characters.
Q2: What is a character variable?
A character variable is a variable that can store a single character.
Q3: What is the index operator?
The index operator ([]) is used to access a single element in an array or a string.
Q4: How do I convert a std::string to a char*?
You can use the c_str() method of the std::string class to convert a std::string to a const char*. Here's an example:
std::string myString = "Hello";
const char* myCharPtr = myString.c_str(); // Converts the string to a char*
Q5: Can I use single quotes to represent a string literal?
No, single quotes represent a single character, while double quotes represent a string literal.
Conclusion
The "invalid conversion from char* to char" error can be frustrating, but it's easy to fix once you know the solutions. By using single quotes, the index operator, or the std::string class, you can avoid this error and write clean and efficient C++ code.
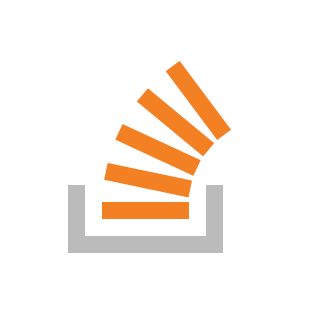