If you are a C# developer, you may have encountered the InvalidOperationException
error with the message "Collection was modified; enumeration operation may not execute". This error occurs when you try to modify a collection while iterating over it. In this guide, we will explain the causes of this error and provide solutions to fix it.
Causes of the Error
There are several reasons why you may encounter the "Collection was modified" error in your C# program:
1. Modifying a Collection while Iterating Over it
One of the most common causes of this error is modifying a collection while iterating over it. For example, you may be using a foreach
loop to iterate over a list and then add or remove items from the same list within the loop.
2. Multiple Threads Modifying the Same Collection
Another reason why you may encounter this error is when multiple threads are modifying the same collection at the same time. This can cause synchronization issues and result in the "Collection was modified" error.
3. Using an Enumerator after Modifying a Collection
If you modify a collection and then try to use an enumerator to iterate over it, you will encounter the "Collection was modified" error.
Solutions to Fix the Error
Here are some solutions to fix the "Collection was modified" error in your C# program:
1. Use a Copy of the Collection
To avoid modifying a collection while iterating over it, you can create a copy of the collection and iterate over the copy instead. This way, you can modify the original collection without causing any issues.
List<int> list = new List<int>() { 1, 2, 3 };
List<int> copy = new List<int>(list);
foreach (int item in copy)
{
list.Remove(item);
}
2. Use a Synchronized Collection
If multiple threads are modifying the same collection, you can use a synchronized collection to avoid synchronization issues. The ConcurrentBag
class in the System.Collections.Concurrent
namespace is an example of a synchronized collection.
ConcurrentBag<int> bag = new ConcurrentBag<int>() { 1, 2, 3 };
Parallel.ForEach(bag, (item) =>
{
bag.Add(item + 1);
});
3. Use a Lock to Synchronize Access to the Collection
Another way to avoid synchronization issues when multiple threads are modifying the same collection is to use a lock to synchronize access to the collection. You can use the lock
keyword to create a critical section that only one thread can access at a time.
List<int> list = new List<int>() { 1, 2, 3 };
object syncObject = new object();
Parallel.ForEach(list, (item) =>
{
lock (syncObject)
{
list.Add(item + 1);
}
});
4. Use a Different Enumerator after Modifying a Collection
If you modify a collection and then try to use an enumerator to iterate over it, you will encounter the "Collection was modified" error. To avoid this, you can create a new enumerator after modifying the collection.
List<int> list = new List<int>() { 1, 2, 3 };
IEnumerator<int> enumerator = list.GetEnumerator();
list.Add(4);
enumerator = list.GetEnumerator();
while (enumerator.MoveNext())
{
Console.WriteLine(enumerator.Current);
}
FAQ
Q1. What is the "Collection was modified" error?
A1. The "Collection was modified" error is an InvalidOperationException
that occurs when you try to modify a collection while iterating over it.
Q2. What causes the "Collection was modified" error?
A2. The "Collection was modified" error can be caused by modifying a collection while iterating over it, using an enumerator after modifying a collection, or having multiple threads modify the same collection at the same time.
Q3. How can I fix the "Collection was modified" error?
A3. You can fix the "Collection was modified" error by using a copy of the collection, using a synchronized collection, using a lock to synchronize access to the collection, or creating a new enumerator after modifying the collection.
Q4. Can I modify a collection while using a for
loop?
A4. Yes, you can modify a collection while using a for
loop as long as you are not adding or removing items from the collection.
Q5. How can I prevent multiple threads from modifying the same collection?
A5. You can prevent multiple threads from modifying the same collection by using a synchronized collection or by using a lock to synchronize access to the collection.
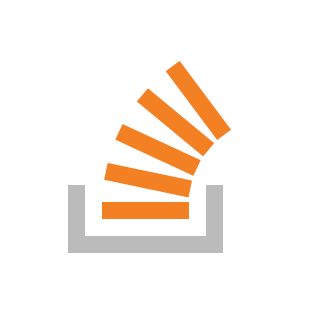