The error "list to dictionary function for fantasy game inventory" likely refers to a problem with converting a list of items in a fantasy game inventory to a dictionary. Here is one way to fix this issue:
- First, create an empty dictionary to store the inventory items.
- Iterate through the list of items using a for loop.
- For each item in the list, add the item as a key to the dictionary and set the value to 1.
- If an item is already in the dictionary, increment the value by 1.
- Return the dictionary
Here's an example of the code:
def list_to_dict(inventory):
inventory_dict = {}
for item in inventory:
if item in inventory_dict:
inventory_dict[item] += 1
else:
inventory_dict[item] = 1
return inventory_dict
You can call this function by passing a list of inventory items as an argument.
For example:
inventory = ["sword", "armor", "shield", "sword", "armor", "potion"]
inventory_dict = list_to_dict(inventory)
print(inventory_dict)
This will print {"sword": 2, "armor": 2, "shield": 1, "potion": 1}
This code will convert the list of items into a dictionary where each key is an item from the list, and the value is the number of times that item appears in the list. This can be useful for keeping track of the number of each item in the inventory.
Another approach that you can use to fix this error is to use the collections.Counter
method. The collections
module in Python provides alternatives to built-in types that can be more efficient than the alternatives. The Counter
method is a dict subclass for counting hashable objects.
You can use it to convert a list to a dictionary where the keys are the items in the list and the values are the number of times the item appears in the list.
Here's an example:
from collections import Counter
def list_to_dict(inventory):
inventory_dict = Counter(inventory)
return inventory_dict
inventory = ["sword", "armor", "shield", "sword", "armor", "potion"]
inventory_dict = list_to_dict(inventory)
print(inventory_dict)
This will also print {"sword": 2, "armor": 2, "shield": 1, "potion": 1}
This approach is more concise, and it uses the power of the built-in collections
module to simplify the process of counting the items in the list.
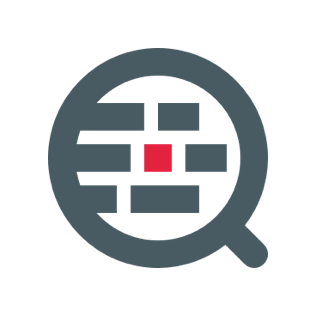
Frequently Asked Questions About The Error
Q: What causes the error? A: The error is caused when there is a problem with converting a list of items in a fantasy game inventory to a dictionary. This can happen if the code is not properly written to iterate through the list, add items as keys to the dictionary, and set the values to the number of times the item appears in the list.
Q: How can I fix the error?
There are a few ways to fix the error. One approach is to use a for loop to iterate through the list, add items as keys to the dictionary, and increment the value for each item that already exists in the dictionary. Another approach is to use the collections.Counter
method to convert the list to a dictionary where the keys are the items in the list and the values are the number of times the item appears in the list.
Q: How can I prevent this error from happening again?
To prevent this error from happening again, make sure to thoroughly test your code before deploying it. Also, make sure you understand the logic behind converting a list to a dictionary and the specific requirements of your fantasy game inventory.
Q: How Can I check if the conversion is done correctly?
You can check the conversion by comparing the input list and the output dictionary. You can check if each item in the list is present in the dictionary and the value of the item in the dictionary is the number of times it appears in the list.