The error message "subscript indices must either be real positive integers or logicals" in MATLAB occurs when you are trying to access an element of an array using an index that is not a positive integer or logical value. This can happen in several ways:
- You might be trying to access an element using a non-integer value, such as a decimal or a variable that contains a decimal.
- You might be trying to access an element using a negative integer, which is not allowed in MATLAB.
- You might be trying to access an element using a logical value that is not true or false.
- You might be trying to access an element using an index that is out of range for the array.
To fix the error, you need to make sure that the indices you are using to access the elements of the array are valid. This can be done by:
- Using the round() function to convert decimal values to integers before using them as indices.
- Using the abs() function to convert negative integers to positive integers before using them as indices.
- Using logical indexing (i.e., using a logical array to index into another array) instead of using logical values directly as indices.
- Checking the size of the array and making sure that the indices you are using are within the valid range.
For example:
A=[1 2 3; 4 5 6; 7 8 9];
index =1.5;
index = round(index);
A(index)
This will access the element in the array A with index 1 and it will not give the error message.
Another example:
A=[1 2 3; 4 5 6; 7 8 9];
index =-2;
index = abs(index);
A(index)
This will access the element in the array A with index 2 and it will not give the error message.
Additionally, when using logical indexing, you can use the find() function to convert logical values to their corresponding indices:
A=[1 2 3; 4 5 6; 7 8 9];
logical_index = A > 2;
index = find(logical_index);
A(index)
This will return all elements of A that are greater than 2.
Lastly, if you have any doubt about the size of the array and the indices you are using, you can use the size() function to check the size of the array and the length() function to check the number of elements along a specific dimension. This will allow you to make sure that the indices you are using are within the valid range for the array:
A=[1 2 3; 4 5 6; 7 8 9];
[m,n] = size(A);
index = m + 1;
if index > m*n
disp("Index out of range")
else
A(index)
end
This will check if the index is out of range and if it is it will display a message "Index out of range" otherwise it will access the element in the array A with the specified index.
By following these steps, you should be able to fix the "subscript indices must either be real positive integers or logicals" error in MATLAB.
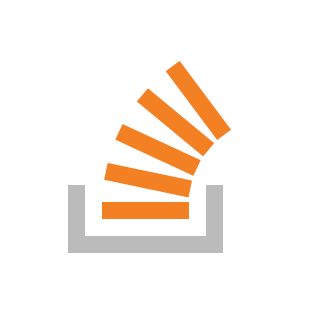
Frequently Asked Questions About The Error
What does the error message "subscript indices must either be real positive integers or logicals" mean?
This error message occurs in MATLAB when you are trying to access an element of an array using an index that is not a positive integer or logical value.
What are some common causes of this error?
Some common causes of this error include using a non-integer value as an index, using a negative integer as an index, using a logical value that is not true or false as an index, and using an index that is out of range for the array.
How can I fix this error?
To fix this error, you need to make sure that the indices you are using to access the elements of the array are valid. This can be done by using the round() function to convert decimal values to integers, using the abs() function to convert negative integers to positive integers, using logical indexing, and checking the size of the array and making sure that the indices you are using are within the valid range.
Can I use negative indices to access elements in an array?
No, in MATLAB you cannot use negative indices to access elements in an array. Indices must be positive integers or logical values.
Can I use decimal values as indices?
No, in MATLAB you cannot use decimal values as indices. Indices must be positive integers or logical values. You can use the round() function to convert decimal values to integers before using them as indices.
How can I use logical indexing to access elements in an array?
You can use the find() function to convert logical values to their corresponding indices, and then use those indices to access the elements in the array. For example, using the statement "index = find(A > 2)" will return the indices of all elements in the array A that are greater than 2. You can then use this "index" variable to access those elements in the array.