In React, you can pass a component as a prop to another component. This can be useful for creating reusable components that can be customized with different implementations for specific parts of the UI.
To pass a component as a prop in React, you will need to define a prop on the receiving component with a type of React.ElementType
or a type that is a function that returns a React element. Then, you can pass a component to this prop when you use the receiving component.
Here is an example of a component that receives a component as a prop:
import React from 'react';
type Props = {
title: string,
Content: React.ElementType
};
function MyCustomCard(props: Props) {
const { title, Content } = props;
return (
<div className="custom-card">
<h2 className="custom-card__title">{title}</h2>
<div className="custom-card__content">
<Content />
</div>
</div>
);
}
In this example, the MyCustomCard
component receives a Content
prop that is a component. The Content
component is rendered inside the custom-card__content
element.
To use this component and pass a component as the Content
prop, you can do the following:
import React from 'react';
function MyContent() {
return <p>This is the content for the custom card</p>;
}
function App() {
return (
<MyCustomCard title="My Custom Card" Content={MyContent} />
);
}
In this example, the MyContent
component is passed as the Content
prop to the MyCustomCard
component. When the MyCustomCard
component is rendered, it will render the MyContent
component inside the custom-card__content
element.
What Is Passing a Component as a Prop in React?
Passing a component as a prop in React means that you pass a reference to a component to another component, which can then use that reference to render the passed component. This allows you to reuse components and build more complex and dynamic user interfaces.
Common Questions and Answers Related to Pass Component as Prop in React
Why would I want to pass a component as a prop in React?
Passing components as props in React allows you to reuse code and create more dynamic and flexible components. For example, you might want to pass a different button component to a form depending on the context in which the form is being used. Or, you might want to pass a custom component to a list to render each item in the list.
What are some best practices for passing components as props in React?
Some best practices for passing components as props in React include:
- Make sure the component being passed as a prop is designed to be used in this way. It should accept props and be able to render itself based on those props.
- Use descriptive prop names to make it clear what the purpose of the prop is.
- Be mindful of the hierarchy of components. Make sure the structure of your components makes sense and is easy to understand.
- Avoid overusing props for data that doesn't need to be passed down. Use state or other means of storing data where appropriate.
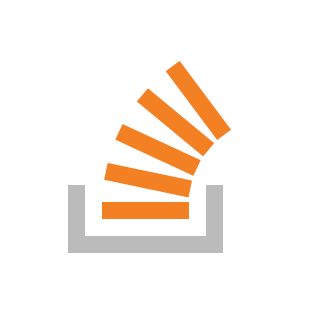