In Android development, managing the lifecycle of an activity is crucial for building efficient and user-friendly applications. There are cases when you need to perform a stop of an activity that won't resume, either due to user interactions or the app's internal logic. In this guide, we'll discuss exclusive tips and tricks to efficiently handle such scenarios.
Table of Contents
Understanding Activity Lifecycle
Before diving into the tips and tricks, it's essential to understand the activity lifecycle in Android. An activity goes through several states during its lifecycle, including onCreate(), onStart(), onResume(), onPause(), onStop(), and onDestroy(). By understanding these states and the transitions between them, you can better manage the resources and improve your app's performance.
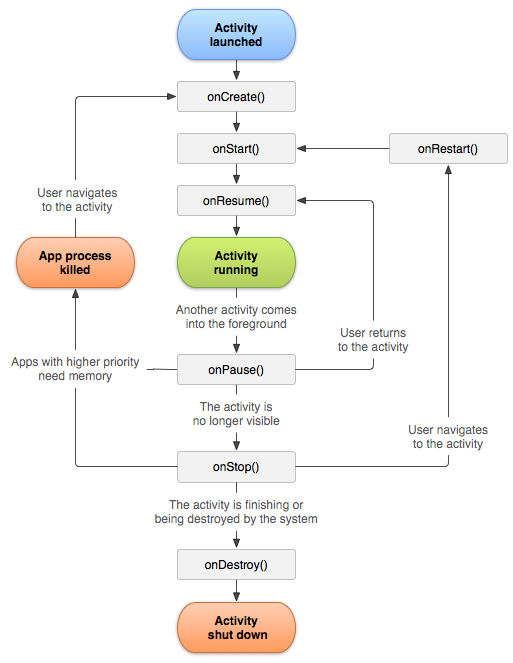
Exclusive Tips for Efficiently Stopping an Activity
Here are some exclusive tips and tricks to efficiently perform a stop of an activity that won't resume:
1. Override onStop() and onDestroy() Methods
Overriding the onStop() and onDestroy() methods allows you to perform cleanup tasks and release resources when your activity is stopped or destroyed. This helps improve your app's performance and reduces the chances of memory leaks.
@Override
protected void onStop() {
// Perform cleanup tasks here
super.onStop();
}
@Override
protected void onDestroy() {
// Release resources here
super.onDestroy();
}
2. Use finish() Method
If you're sure that the activity won't be resumed, you can use the finish() method to destroy it immediately. This method takes care of stopping the activity and removing it from the back stack, ensuring that it won't be resumed.
// Stop the activity and remove it from the back stack
finish();
3. Utilize the noHistory Attribute
Another way to ensure that an activity won't be resumed after being stopped is to set the noHistory
attribute in the <activity>
element of your AndroidManifest.xml file. When this attribute is set to true
, the activity will be removed from the back stack as soon as it's stopped.
<activity
android:name=".MyActivity"
android:noHistory="true">
<!-- ... -->
</activity>
FAQs
1. What is the purpose of the onStop() method in the activity lifecycle?
The onStop()
method is called when the activity is no longer visible to the user. This can happen when the activity is being destroyed, or another activity is being resumed and covering it. It's a good place to perform cleanup tasks, such as releasing resources, unregistering receivers, or stopping services.
2. What is the difference between onStop() and onDestroy()?
The onStop()
method is called when the activity becomes invisible to the user, while the onDestroy()
method is called when the activity is being destroyed. The onDestroy()
method is the final call in the activity lifecycle, and it's where you should release all resources and perform any final cleanup tasks.
3. When should I use the finish() method?
You should use the finish()
method when you want to stop an activity and ensure it won't be resumed. This method stops the activity and removes it from the back stack, preventing it from being resumed by the user.
4. Can I use the noHistory attribute for all activities in my app?
Using the noHistory
attribute for all activities in your app is not recommended, as it may lead to unexpected behavior and a poor user experience. This attribute should only be used for specific activities that you want to ensure won't be resumed after being stopped.
5. How can I ensure that the onStop() and onDestroy() methods are called when stopping an activity?
To ensure that the onStop()
and onDestroy()
methods are called when stopping an activity, you should always call the super
implementation of these methods. This ensures that the Android system can perform the necessary internal operations to stop and destroy the activity.
@Override
protected void onStop() {
// Perform cleanup tasks here
super.onStop();
}
@Override
protected void onDestroy() {
// Release resources here
super.onDestroy();
}