Here is an example of how to remove the last element from a vector called "ticketlist" in C++:
#include <vector>
int main() {
std::vector<int> ticketlist{1, 2, 3, 4, 5};
// remove the last element
ticketlist.pop_back();
// print the updated vector
for (int i : ticketlist) {
std::cout << i << " ";
}
return 0;
}
In this example, the pop_back()
function is used to remove the last element from the "ticketlist" vector. This function does not take any arguments and does not return a value. After calling this function, the last element is removed from the vector, and the size of the vector is decreased by one. The for-each loop is used to print the updated vector.
You can also use the iterator to remove an element from a vector.
ticketlist.erase(ticketlist.end()-1);
This will also remove the last element of the vector.
It's worth noting that in both case the element will be removed from the memory and the vector's size will decrease but it doesn't guarantee that the memory will be freed.
You can also use the std::vector::back()
function to get a reference to the last element of the vector before removing it:
int last_element = ticketlist.back();
ticketlist.pop_back();
This way, you can save the last element in a variable before removing it from the vector.
It's worth noting that if the vector is empty and you try to call pop_back()
or back()
on it, it will result in an error, so it's a good practice to check if the vector is empty before attempting to remove the last element.
if(!ticketlist.empty()){
ticketlist.pop_back();
}
It's also worth mentioning that removing the last element from a vector with the pop_back()
function is an O(1) operation, meaning it has a constant-time complexity and it is a fast operation.
Related Issues:
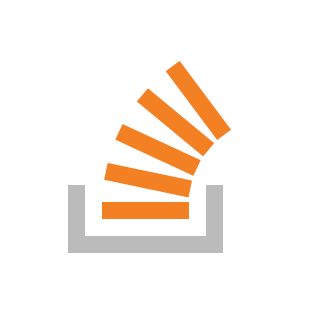
Frequently Asked Questions About "Remove The Last Element From Vector Ticketlist"
How do I know if the vector is empty before trying to remove the last element?
You can use the std::vector::empty()
function to check if a vector is empty. This function returns a Boolean value indicating whether the vector is empty or not. An example would be if(!ticketlist.empty())
2. Can I remove an element other than the last one using the pop_back()
function?
No, the pop_back()
function can only be used to remove the last element of a vector. If you want to remove an element other than the last one, you can use the std::vector::erase()
function, which takes an iterator pointing to the element to be removed as its argument.
3. Will the memory be freed after removing the last element from a vector?
Removing an element from a vector does not guarantee that the memory will be freed. The memory allocated for the vector is managed by the vector itself and it is designed to be efficient in terms of memory usage. If you want to free the memory you should use a different data structure.
4. Is there any performance difference between pop_back()
and erase(end()-1)
?
Both pop_back()
and erase(end()-1)
will remove the last element of a vector, but pop_back()
is generally faster because it only needs to update the size of the vector, while erase(end()-1)
needs to shift all the elements after the removed element to fill the gap.
5. Can the last element be removed without using the pop_back()
or erase()
function?
Yes, you can use a combination of the std::vector::back()
and std::vector::pop_back()
function to remove the last element from a vector, as well as the std::vector::erase()
function with an iterator pointing to the last element.
6. How can I remove all elements from the vector?
You can use the std::vector::clear()
function to remove all elements from a vector.
ticketlist.clear();
This will remove all elements from the vector and set its size to 0, but it doesn't guarantee that the memory will be freed.