When working with Python code, you may encounter the error message 'TypeError: 'bool' object is not iterable'. This error usually occurs when you try to iterate over a boolean value (True or False) using a loop or a comprehension. In this guide, we will explore the causes of this error and provide step-by-step solutions on how to resolve it.
Causes of 'TypeError: 'bool' Object is Not Iterable' Error in Python Code
The 'TypeError: 'bool' object is not iterable' error occurs when you try to iterate over a boolean value using a loop or a comprehension. For example, consider the following code snippet:
flag = True
for i in flag:
print(i)
This code will result in the following error message:
TypeError: 'bool' object is not iterable
The error occurs because the bool type is not iterable in Python. You cannot loop over a boolean value.
Resolving 'TypeError: 'bool' Object is Not Iterable' Error in Python Code
To resolve the 'TypeError: 'bool' object is not iterable' error, you need to ensure that you are not trying to iterate over a boolean value. There are several ways to do this:
1. Check the Type of the Variable
Before iterating over a variable, check its type to ensure that it is iterable. You can use the type()
function to check the type of a variable. For example:
flag = True
if type(flag) == bool:
print("Variable is a boolean value")
else:
print("Variable is not a boolean value")
This code will output:
Variable is a boolean value
2. Convert the Boolean Value to an Iterable
If you need to iterate over a boolean value, you can convert it to an iterable using the range()
function. For example:
flag = True
for i in range(int(flag)):
print(i)
This code will output:
0
1
3. Use a List Comprehension
If you need to iterate over a boolean value and create a list, you can use a list comprehension. For example:
flag = True
lst = [i for i in range(int(flag))]
print(lst)
This code will output:
[0, 1]
FAQ
What is the 'bool' type in Python?
The 'bool' type in Python represents the two boolean values True and False.
Why is the 'bool' type not iterable in Python?
The 'bool' type is not iterable in Python because it only has two possible values (True and False).
How can I check the type of a variable in Python?
You can check the type of a variable in Python using the type()
function.
Can I convert a boolean value to an iterable in Python?
Yes, you can convert a boolean value to an iterable in Python using the range()
function.
What is a list comprehension in Python?
A list comprehension is a concise way to create a list in Python. It consists of an expression followed by a for clause and an optional if clause.
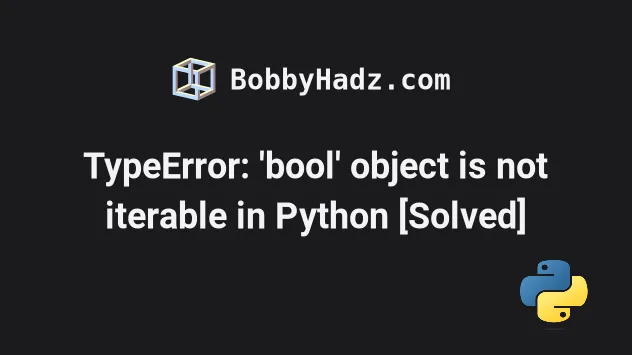