The "TypeError: Cannot read property 'length' of null" error occurs when you try to access the "length" property of an object that is null or undefined. This means that the object is not an instance of an Array or a string, and therefore doesn't have a "length" property. To fix this error, you need to ensure that the object is not null or undefined before accessing its properties.
Here's an example of how to fix the error:
let myArray = [1, 2, 3];
let myObject = null;
if (myArray) {
console.log(myArray.length); // 3
}
if (myObject) {
console.log(myObject.length); // TypeError: Cannot read property 'length' of null
} else {
console.log('myObject is null or undefined');
}
Another way to handle this error is to use type checking before accessing the property. Here's an example using typeof operator:
let myArray = [1, 2, 3];
let myObject = null;
if (typeof myArray !== 'undefined' && myArray !== null) {
console.log(myArray.length); // 3
}
if (typeof myObject !== 'undefined' && myObject !== null) {
console.log(myObject.length); // TypeError: Cannot read property 'length' of null
} else {
console.log('myObject is null or undefined');
}
In the above code, we check if the myArray
and myObject
are not undefined
and null
before accessing their properties.
Alternatively, you can use a try-catch block to handle this error:
let myArray = [1, 2, 3];
let myObject = null;
try {
console.log(myArray.length); // 3
console.log(myObject.length);
} catch (error) {
console.error(error);
console.log('myObject is null or undefined');
}
In this case, if the myObject.length
property throws an error, it will be caught by the catch block, and you can handle it accordingly.
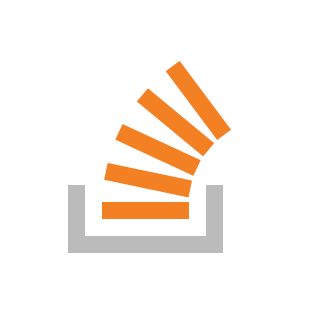
Frequently Asked Questions About The Error
What does the error "TypeError: Cannot read property 'length' of null" mean?
The error message "TypeError: Cannot read property 'length' of null" indicates that you are trying to access a property (in this case "length") of an object that is null
or undefined
, and the property doesn't exist on that object.
Why am I getting this error?
You may get this error when you are trying to access a property of an object that is either null
or undefined
. It could be because the object wasn't properly initialized or the property is not defined for that object.
How do I fix this error?
You can fix this error by either checking if the object is null
or undefined
before accessing its properties or using a try-catch block to handle the error.
Can this error be caused by a missing reference?
Yes, this error can be caused by a missing reference. For example, if you are trying to access an object property that doesn't exist, you will get this error.
Can this error be related to asynchronous programming?
Yes, this error can be related to asynchronous programming if you are trying to access an object property that hasn't been initialized yet. In such cases, it's important to wait for the data to be available before accessing its properties.