Java is an immensely popular, open-source language commonly used for web and mobile applications, but it's also used to create complex computer programs. In this post, we’ll walk you through how to set up a program to simulate a car racing activity using Java. We’ll also go over useful tips and tricks and answer the most frequently asked questions associated with writing this type of program.
Prerequisites
Before we jump into writing code, let’s make sure your environment is set up to work on this project. Here are the prerequisites you’ll need:
- Java JDK – You’ll need this framework in order to develop, compile, and run your Java program.
- Text Editor – You’ll need a modern text editor to write, manage, and edit your code. Our instructions will be based on Atom.
- Programming Fundamentals – You should understand core programming concepts, such as data types, classes, and methods.
Step 1 – Writing the Program
Now you’re ready to start writing your program. Let’s begin by creating a Java class called RacingGame. This class will define the behavior and processes associated with our racing game. Inside this class, you will use the main()
method to start the program.
public class RacingGame {
public static void main (String[] args) {
// program commands go here
}
}
Next, you’ll need to define the necessary elements of the game. This includes the race cars and the track(s) for the cars to race on.
Create a RaceCar
class to define its attributes. The game will support multiple cars with their own unique attributes, such as speed and color. The RaceCar
class should also include methods to access and modify these attributes.
public class RaceCar {
// car attributes
private double speed;
private String color;
//constructors
public RaceCar() {
this.speed = 0;
this.color = "";
}
public RaceCar(double speed, String color) {
this.speed = speed;
this.color = color;
}
// accessor methods
public double getSpeed() {
return this.speed;
}
public String getColor() {
return this.color;
}
// mutator methods
public void setSpeed(double speed) {
this.speed = speed;
}
public void setColor(String color) {
this.color = color;
}
}
Create a RacingTrack
class, which will contain methods related to setting up a racing track and calculating the positions of the cars each lap. The class will define the length of the track, the number of cars racing, and the lap limit.
public class RacingTrack {
// track attributes
private int length;
private int numCars;
private int lapLimit;
// constructors
public RacingTrack() {
this.length = 0;
this.numCars = 0;
this.lapLimit = 0;
}
public RacingTrack(int length, int numCars, int lapLimit) {
this.length = length;
this.numCars = numCars;
this.lapLimit = lapLimit;
}
// accessor methods
public int getLength() {
return this.length;
}
public int getNumCars() {
return this.numCars;
}
public int getLapLimit() {
return this.lapLimit;
}
// mutator methods
public void setLength(int length) {
this.length = length;
}
public void setNumCars(int numCars) {
this.numCars = numCars;
}
public void setLapLimit(int lapLimit) {
this.lapLimit = lapLimit;
}
// calculate positions
public int[] calculateCarPositions(){
return this.positions;
}
}
Step 2 – Compiling your Program
Now that your code is written, it’s time to compile your program. To do this, you’ll use the Java compiler tool javac
. Open your command line and navigate to the directory containing your program’s .java
file, then type in the javac
command, followed by a space and the name of your file.
javac RacingGame.java
Step 3 – Running your Program
Once your program is compiled, it’s time to execute it. To do this, you’ll use the Java Virtual Machine (JVM). Type in the command java
in your command line, followed by a space and the name of your file.
java RacingGame
When you run this command, your car racing game should now be fully functional!
FAQ
Q1. What is the best way to debug my code?
The best way to debug a Java program is to use the built-in debugging features within your IDE (Integrated Development Environment). For example, Eclipse has a powerful debugging feature that allows you to inspect and modify running code, set breakpoints, and step through your code line-by-line.
Q2. What is the purpose of the main()
method?
The main()
method is the starting point of a Java program. It's where the program execution begins. It's also where any command line arguments can be passed in and processed.
Q3. How can I make my program more efficient?
One way to improve the efficiency of your program is to use Java's memory management and garbage collection functions. By managing memory efficiently, you can boost performance and reduce the amount of time it takes to execute your program.
Q4. What are best practices for coding in Java?
For best coding practices, you should strive to write code that is easy to read and understand. Use consistent formatting, include comments in code blocks, and ensure that variables, classes, and methods are named accurately. Additionally, you should always use version control to track changes to your code and make sure you're making use of existing libraries whenever possible.
Q5. What is the name of the language used to develop Java programs?
The language used to develop Java programs is called Java.
Related Links
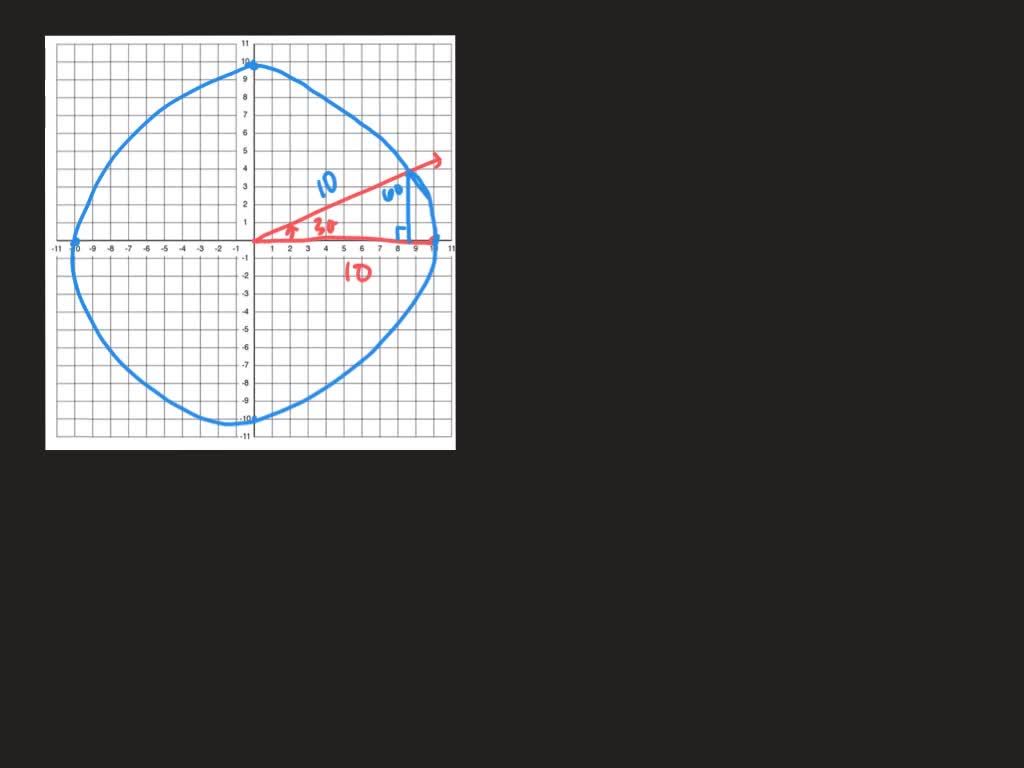