clsx
is a utility function for constructing class names in React. It allows you to combine multiple class names dynamically based on the values of certain conditions.
For example, you might want to include a certain class name if a boolean prop is true
, and a different class name if it is false
. You can use clsx
to combine these class names based on the value of the prop:
import clsx from 'clsx';
function MyComponent(props) {
const className = clsx(
'my-class',
{ 'my-other-class': props.isTrue },
{ 'my-third-class': !props.isTrue }
);
return <div className={className}>Hello World!</div>;
}
In this example, if props.isTrue
is true
, the resulting className
will be 'my-class my-other-class'
. If props.isTrue
is false
, the resulting className
will be 'my-class my-third-class'
. clsx
allows you to easily combine class names like this in a concise and readable way.
What is CLSX React?
clsx
is a utility function for constructing class names in JavaScript. It is not a specific library or framework, but it is often used in projects that make use of the React library, which is a JavaScript library for building user interfaces.
clsx
can be used to conditionally join a list of class names together, allowing you to easily add or remove classes based on certain conditions. It is useful for creating flexible and reusable component styles in React projects.
clsx
is used to join these class names together in a way that allows the component to be easily customized with CSS.
Common Question and a Answers Related to CLSX React
How do I use clsx
in a React component?
To use clsx
in a React component, you will need to install the clsx
package and import it into your component file. Then, you can use the clsx
function to join together a list of class names based on certain conditions.
Here is an example of how to use clsx
in a React component:
import clsx from 'clsx';
function MyComponent(props) {
const { className, isActive } = props;
const componentClasses = clsx(
'my-component',
{ 'my-component--active': isActive },
className
);
return <div className={componentClasses}>{/* component JSX */}</div>;
}
How do I pass additional class names to a React component using clsx
?
To pass additional class names to a React component using clsx
, you can use the className
prop. The value of the className
prop should be a string containing a space-separated list of class names.
Here is an example of how to pass additional class names to a React component using clsx
:
import clsx from 'clsx';
function MyComponent(props) {
const { className, isActive } = props;
const componentClasses = clsx(
'my-component',
{ 'my-component--active': isActive },
className
);
return <div className={componentClasses}>{/* component JSX */}</div>;
}
// Use the component and pass in additional class names
<MyComponent className="my-additional-class another-class" />
Can I use clsx
to toggle a class based on a condition in a React component?
Yes, you can use clsx
to toggle a class based on a condition in a React component. To do this, you can use the object syntax for passing class names to clsx
, where the keys are the class names and the values are boolean expressions that determine whether or not the class should be included.
Here is an example of how to use clsx
to toggle a class based on a condition in a React component:
import clsx from 'clsx';
function MyComponent(props) {
const { isActive } = props;
const componentClasses = clsx(
'my-component',
{ 'my-component--active': isActive }
);
return <div className={componentClasses}>{/* component JSX */}</div>;
}
In this example, the my-component--active
class will be included in the div
element's class
attribute if isActive
is true
, and it will be excluded if isActive
is false
.
Related Links:
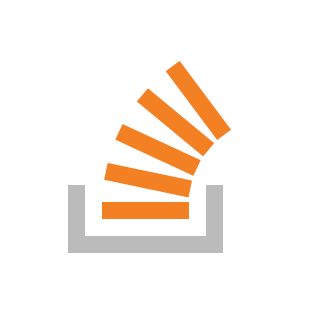
