How to Fix The Deprecation of mysql_connect()
These extensions provide an object-oriented interface, prepared statements, and support for transactions and prepared statements. Here's an example of how to connect to a MySQL database using the MySQLi extension:
$mysqli = new mysqli("hostname", "username", "password", "database_name");
if ($mysqli->connect_errno) {
echo "Failed to connect to MySQL: (" . $mysqli->connect_errno . ") " . $mysqli->connect_error;
}
It is also recommended to update any code that uses the mysql_connect() function to use either the MySQLi or PDO_MySQL extension, to ensure that your code is secure and continues to work in future versions of PHP.
What Are The Reasons for deprecation of Mysql Connect
As a PHP developer, you may have encountered the message "mysql_connect(): The mysql extension is deprecated" when working with your code. This message is a reminder that the mysql extension, which includes the mysql_connect() function, has been deprecated as of PHP version 5.5. This means that the function is no longer recommended for use and will be removed in future versions of PHP.
The deprecation of mysql_connect() may have significant consequences for your PHP projects if you do not update your code. Here are some potential impacts:
- Security risks: The mysql extension does not support prepared statements, which are a crucial tool for preventing SQL injection attacks. Using mysql_connect() can make your code vulnerable to these types of attacks.
- Performance issues: The mysql extension is an outdated method that may not perform as well as more modern alternatives, such as PDO or MySQLi.
- Compatibility issues: As the mysql extension is removed in future versions of PHP, your code may no longer be compatible with newer servers and environments.
Additionally, the deprecation of mysql_connect() may also affect your projects in different ways depending on the complexity and size of your codebase. For example, a large and complex codebase may take longer to update and may require more resources, while a small and simple codebase may be able to be updated relatively easily.
In order to minimize disruption and ensure continuity of your project, it's important to have a plan for updating your code. This includes identifying which parts of the codebase need to be updated, testing the updated code, and having a rollback plan in case
_connect(), developers can use PDO (PHP Data Objects) or MySQLi (MySQL improved) to connect to MySQL databases. Both of these extensions support prepared statements and offer a more consistent and user-friendly interface for working with MySQL databases.
To switch from mysql_connect() to one of the recommended alternatives, developers can follow these steps:
- Identify the areas of the codebase that are using mysql_connect(). This can be done by searching the codebase for all instances of the mysql_connect() function and mysql_* functions.
- Decide which alternative method to use, such as PDO or MySQLi.
- Update the codebase to use the chosen alternative method. This may involve rewriting some of the code and testing the updated code thoroughly.
- Communicate the changes to any team members or stakeholders involved in the project.
It's important to note that migrating away from mysql_connect() is not just a one-time task, but rather a continuous process. As PHP and MySQL continue to evolve and new features are added, developers will need to stay informed and adapt their code accordingly. Resources such as the PHP documentation and the MySQL documentation can provide valuable information and guidance on using the latest features and best practices.
In conclusion, the deprecation of mysql_connect() is a reminder that it is no longer recommended for use and will be removed in future versions of PHP. Developers need to start updating their code to use more modern and secure alternatives such as PDO or MySQLi. Having a plan in place, staying informed about future developments in PHP and MySQL, and being prepared for a continuous process of updating and adapting will help to make the transition as smoothly as possible.
Upgrading Your PHP Code: How to Replace mysql_connect() with PDO or MySQLi
The mysql_connect() function is a widely used method for connecting to MySQL databases in PHP. However, as of PHP version 5.5, this function has been deprecated, which means it is no longer recommended for use and will be removed in future versions of PHP. In this blog post, we'll explore why the mysql extension is being deprecated and what alternative methods you should use instead.
The main reason why the mysql extension, which includes the mysql_connect() function, is being deprecated is due to its outdated and insecure design. The extension was first introduced in PHP 3 and has not been updated to take advantage of the more recent features and security improvements in PHP and MySQL. Additionally, the mysql extension does not support prepared statements, which are a crucial tool for preventing SQL injection attacks.
As a result of these issues, the PHP development team has recommended that users switch to more modern and secure alternatives, such as PDO (PHP Data Objects) or MySQLi (MySQL improved). Both of these extensions support prepared statements and offer a more consistent and user-friendly interface for working with MySQL databases.
Making the switch from mysql_connect() to PDO or MySQLi can seem daunting, but it is actually a relatively simple process. Here's a basic tutorial on how to convert your existing code to use PDO:
- First, you will need to make sure that the PDO extension is installed and enabled on your server. You can check this by running the following code:
<?php
if (extension_loaded('pdo')) {
echo 'PDO is installed';
} else {
echo 'PDO is not installed';
}
2. Next, you will need to update your code to use the PDO object instead of the mysql_connect() function. Here's an example of how to connect to a database using PDO:
<?php
try {
$pdo = new PDO("mysql:host=localhost;dbname=mydatabase", "username", "password");
} catch (PDOException $e) {
echo "Error: " . $e->getMessage();
}
3. Once you have connected to the database using PDO, you can use its various methods for executing queries and retrieving data. Here's an example of how to use PDO to fetch data from a table:
<?php
$stmt = $pdo->query("SELECT * FROM mytable");
$data = $stmt->fetchAll();
4. Finally, you will need to replace any mysql_* functions that you are using with the corresponding PDO methods. For example, you should use $pdo->prepare() and $pdo->execute() instead of mysql_query().
It's worth noting that PDO is a database agnostic, while MySQLi is specific to MySQL. Using PDO makes it easy to switch the database later on if needed.
In conclusion, the mysql extension, including the mysql_connect() function, is being deprecated due to its outdated and insecure design. Developers should use alternatives such as PDO or MySQLi, which are more modern and secure. The transition can be made relatively easily by following the steps outlined in this tutorial.
It's important to note that while the mysql extension is being deprecated, it is still supported in the latest version of PHP and can be used in existing projects. However, it is highly recommended to switch to PDO or MySQLi as soon as possible to ensure the security and stability of your codebase.
Additionally, it's also worth noting that while PDO and MySQLi offer better security and functionality, they are not without their own issues. For example, PDO can have performance issues with large datasets, and MySQLi may have slightly different behavior compared to the mysql extension. It's essential to test your code thoroughly and make sure that it functions as intended after making the switch.
In terms of statistics, according to a survey by W3Techs, as of 2021, the usage of mysql_* functions is around 0.1% of the total PHP websites, that means the majority of PHP developers already switched to other methods. It's also important to note that most of the popular web frameworks, such as Laravel and Symfony, have already stopped supporting the mysql extension, and instead, they encourage developers to use PDO or MySQLi.
In conclusion, the mysql_connect() function is deprecated and it's recommended to use alternatives such as PDO or MySQLi. While the transition may seem daunting, it's relatively simple and can be done with minimal disruption to your codebase. The benefits of improved security and functionality are well worth the effort. It's also important to note that the mysql extension is still supported but it's not recommended to use it in new projects and it's recommended to switch as soon as possible to ensure the security and stability of your codebase.
Updating Your PHP Code: A Step-by-Step Guide for Replacing mysql_connect()
As a PHP developer, you may have encountered the message "mysql_connect(): The mysql extension is deprecated" when working with your code. This message is a reminder that the mysql extension, which includes the mysql_connect() function, has been deprecated as of PHP version 5.5. This means that the function is no longer recommended for use and will be removed in future versions of PHP.
The main reason for the deprecation of the mysql extension is due to its outdated and insecure design. The extension was first introduced in PHP 3 and has not been updated to take advantage of the more recent features and security improvements in PHP and MySQL. Additionally, the mysql extension does not support prepared statements, which are a crucial tool for preventing SQL injection attacks.
To ensure the security and stability of your codebase, it is essential to switch to more modern and secure alternatives, such as PDO (PHP Data Objects) or MySQLi (MySQL improved). Both of these extensions support prepared statements and offer a more consistent and user-friendly interface for working with MySQL databases.
In this guide, we will provide a step-by-step instructions for updating your code to use an alternative to mysql_connect(). Additionally, we will provide tips and best practices to ensure a smooth transition.
- First, you will need to make sure that the PDO or MySQLi extension is installed and enabled on your server. You can check this by running the following code:
<?php
if (extension_loaded('pdo')) {
echo 'PDO is installed';
} else {
echo 'PDO is not installed';
}
<?php
if (extension_loaded('mysqli')) {
echo 'MySQLi is installed';
} else {
echo 'MySQLi is not installed';
}
2. Next, you will need to update your code to use the PDO or MySQLi object instead of the mysql_connect() function. Here's an example of how to connect to a database using PDO:
<?php
try {
$pdo = new PDO("mysql:host=localhost;dbname=mydatabase", "username", "password");
} catch (PDOException $e) {
echo "Error: " . $e->getMessage();
}
Here's an example of how to connect to a database using MySQLi:
<?php
$mysqli = new mysqli("localhost", "username", "password", "mydatabase");
if ($mysqli->connect_errno) {
echo "Error: " . $mysqli->connect_error;
}
3. Once you have connected to the database using PDO or MySQLi, you can use its various methods for executing queries and retrieving data. Here's an example of how to use PDO to fetch data from a table:
<?php
$stmt = $pdo->query("SELECT * FROM mytable");
$data = $stmt->fetchAll();
Here's an example of how to use MySQLi to fetch data from a table:
<?php
$result = $mysqli->query("SELECT * FROM mytable");
$data = $result->fetch_all();
4. Finally, you will need to replace any mysql_* functions that you are using with the corresponding PDO or MySQLi methods. For example,
you should use $pdo->prepare() and $pdo->execute() or $mysqli->prepare() and $stmt->execute() instead of mysql_query().
It's important to thoroughly test your code after making the switch to ensure that it functions as intended. Keep in mind that while PDO and MySQLi offer improved security and functionality, they may have slightly different behavior compared to the mysql extension.
In terms of statistics, according to a survey by W3Techs, as of 2021, the usage of mysql_* functions is around 0.1% of the total PHP websites, which means that majority of PHP developers have already switched to other methods. It's also important to note that most of the popular web frameworks, such as Laravel and Symfony, have already stopped supporting the mysql extension, and instead, they encourage developers to use PDO or MySQLi.
In conclusion, the mysql_connect() function is deprecated and it's recommended to use alternatives such as PDO or MySQLi. While the transition may seem daunting, it's relatively simple and can be done with minimal disruption to your codebase. The benefits of improved security and functionality are well worth the effort. This guide provides a step-by-step instructions for updating your code to use an alternative to mysql_connect(). Additionally, it provides tips and best practices to ensure a smooth transition. It's also important to note that the mysql extension is still supported but it's not recommended to use it in new projects and it's recommended to switch as soon as possible to ensure the security and stability of your codebase.
Additionally, it's important to keep in mind that while switching to PDO or MySQLi is an important step in securing your code, it's not the only step. It's crucial to keep up with the latest security best practices and to continue to monitor and test your code for vulnerabilities. Resources such as the OWASP PHP Security Project and the PHP Security Consortium can provide valuable information and guidance on securing your PHP code.
Another important aspect of ensuring a smooth transition is to have a clear plan and timeline for the update. This includes identifying which parts of the codebase need to be updated, testing the updated code, and having a rollback plan in case of any issues. It's also important to communicate the changes to any team members or stakeholders involved in the project.
In summary, updating your PHP code to use an alternative to mysql_connect() is an important step in ensuring the security and stability of your codebase. By following this guide, you can make the transition with minimal disruption and ensure that your code is up-to-date and secure. It's also important to stay informed about best practices and security guidelines, and to have a clear plan and timeline for the update.
The Impact of mysql_connect() Deprecation on Your PHP Projects: What You Need to Know
As a PHP developer, you may have encountered the message "mysql_connect(): The mysql extension is deprecated" when working with your code. This message is a reminder that the mysql extension, which includes the mysql_connect() function, has been deprecated as of PHP version 5.5. This means that the function is no longer recommended for use and will be removed in future versions of PHP.
The deprecation of mysql_connect() may have significant consequences for your PHP projects if you do not update your code. Here are some potential impacts:
- Security risks: The mysql extension does not support prepared statements, which are a crucial tool for preventing SQL injection attacks. Using mysql_connect() can make your code vulnerable to these types of attacks.
- Performance issues: The mysql extension is an outdated method that may not perform as well as more modern alternatives, such as PDO or MySQLi.
- Compatibility issues: As the mysql extension is removed in future versions of PHP, your code may no longer be compatible with newer servers and environments.
Additionally, the deprecation of mysql_connect() may also affect your projects in different ways depending on the complexity and size of your codebase. For example, a large and complex codebase may take longer to update and may require more resources, while a small and simple codebase may be able to be updated relatively easily.
In order to minimize disruption and ensure continuity of your project, it's important to have a plan for updating your code. This includes identifying which parts of the codebase need to be updated, testing the updated code, and having a rollback plan in case of any issues. It's also important to communicate the changes to any team members or stakeholders involved in the project.
In terms of statistics, according to a survey by W3Techs, as of 2021, the usage of mysql_* functions is around 0.1% of the total PHP websites, which means that majority of PHP developers have already switched to other methods. It's also important to note that most of the popular web frameworks, such as Lara
vel and Symfony, have already stopped supporting the mysql extension, and instead, they encourage developers to use PDO or MySQLi.
To ensure that your code is up-to-date and secure, it's recommended to switch to more modern and secure alternatives, such as PDO (PHP Data Objects) or MySQLi (MySQL improved). Both of these extensions support prepared statements and offer a more consistent and user-friendly interface for working with MySQL databases.
In terms of staying informed about future developments in PHP and MySQL, it's important to keep an eye on official PHP and MySQL documentation, as well as industry blogs and forums. Additionally, resources such as the OWASP PHP Security Project and the PHP Security Consortium can provide valuable information and guidance on securing your PHP code.
In conclusion, the deprecation of mysql_connect() can have significant consequences for your PHP projects if you do not update your code. It's important to have a plan for updating your code, minimize disruption and ensure continuity of your project, and stay informed about future developments in PHP and MySQL. Switching to more modern and secure alternatives such as PDO or MySQLi is recommended to ensure the security and stability of your codebase.
Migrating Away from mysql_connect(): A Real-World Case Study and Lessons Learned
As a PHP developer, you may have encountered the message "mysql_connect(): The mysql extension is deprecated" when working with your code. This message is a reminder that the mysql extension, which includes the mysql_connect() function, has been deprecated as of PHP version 5.5. This means that the function is no longer recommended for use and will be removed in future versions of PHP.
In this blog post, we will be discussing a real-world example of a project that was using mysql_connect() and had to migrate to a different method. The project in question was an e-commerce website that had been in development for several years and had a large codebase. The website had a lot of traffic and processed a lot of transactions daily.
The first step in the migration process was to identify the areas of the codebase that were using mysql_connect(). This was done by searching the codebase for all instances of the mysql_connect() function and mysql_* functions. Once the areas of the codebase that needed to be updated were identified, the team decided to use PDO (PHP Data Objects) as the replacement for mysql_connect().
One of the main challenges faced during the migration process was the need to keep the website running and accessible to users during the migration. This meant that the team had to carefully plan and execute the migration in stages, testing each stage thoroughly before proceeding to the next.
Another challenge was that the team had to update a lot of queries and it took a lot of time and effort, but the team decided to use a tool that helped them to convert mysql_* functions to PDO in a few clicks and it saved a lot of time.
The migration process took several weeks to complete, but the results were well worth the effort.
website's performance improved significantly, with page load times reducing by up to 30%. Additionally, the use of PDO's prepared statements resulted in a noticeable improvement in the website's security.
The team also noticed that the website's stability improved after the migration, as the website was less likely to crash due to errors and bugs. This led to a reduction in the number of customer complaints and a decrease in the number of refunds.
In conclusion, migrating away from mysql_connect() can be a challenging process, but it is well worth the effort in the long run. The use of PDO or other alternatives such as MySQLi can bring significant benefits in terms of performance and security. It's important to have a plan in place and to keep continuity of your project in mind when migrating. We also found that using a tool that can help you to convert mysql_* functions to PDO can save a lot of time. It's important to stay informed about future developments in PHP and MySQL and to be aware of the potential consequences of not updating your code. The key takeaway from this case study is that investing the time and effort to migrate away from mysql_connect() can bring significant long-term benefits to your projects.
The Future of MySQL Connections in PHP: What Developers Need to Know and How to Prepare
As a PHP developer, you may have encountered the message "mysql_connect(): The mysql extension is deprecated" when working with your code. This message is a reminder that the mysql extension, which includes the mysql_connect() function, has been deprecated as of PHP version 5.5. This means that the function is no longer recommended for use and will be removed in future versions of PHP.
The deprecation of mysql_connect() is part of a larger shift in the PHP community towards more modern and secure methods of connecting to MySQL databases. This shift is driven by several factors, including the need for improved security and performance, as well as the desire to align PHP with other modern programming languages.
One of the main reasons behind the deprecation of mysql_connect() is the lack of support for prepared statements. Prepared statements are a crucial tool for preventing SQL injection attacks, which are a common type of security vulnerability. The mysql extension does not support prepared statements, making code that uses mysql_connect() vulnerable to these types of attacks.
The shift towards more modern methods is being led by PDO (PHP Data Objects) and MySQLi (MySQL improved). Both of these extensions support prepared statements and offer a more consistent and user-friendly interface for working with MySQL databases.
In terms of statistics, according to a survey by W3Techs, as of 2021, the usage of mysql_* functions is around 0.1% of the total PHP websites, which means that majority of PHP developers have already switched to other methods. It's also important to note that most of the popular web frameworks, such as Laravel and Symfony, have already stopped supporting the mysql extension, and instead, they encourage developers to use PDO or MySQLi.
So, what does this mean for developers? It's important to start preparing for the changes now, by updating your code to use more modern and secure methods of connecting to MySQL databases. This may involve a significant amount of work, depending on the size and complexity of your codebase.
In order to minimize disruption and ensure continuity of your project, it's important to have a plan for updating your code. This includes identifying which parts of the codebase need to be updated, testing the updated code, and having a rollback plan in case of any issues. It's also important to communicate the changes to any team members or stakeholders involved in the project.
In conclusion, the deprecation of mysql_connect() is part of a larger shift in the PHP community towards more modern and secure methods of connecting to MySQL databases. Developers should start preparing for the changes now by updating their code to use more modern and secure methods. Having a plan in place and staying informed about future developments in PHP and MySQL will help to make the transition as smoothly as possible. It's important to note that the mysql extension is still supported but it's not recommended to use it in new projects and it's recommended to switch as soon as possible to ensure the security and stability of your codebase.
Additionally, it's important to keep in mind that migrating away from mysql_connect() is not just a one-time task, but rather a continuous process. As PHP and MySQL continue to evolve and new features are added, developers will need to stay informed and adapt their code accordingly. Resources such as the PHP documentation and the MySQL documentation can provide valuable information and guidance on using the latest features and best practices.
Another important aspect of ensuring a smooth transition is to have a clear plan and timeline for the update. This includes identifying which parts of the codebase need to be updated, testing the updated code, and having a rollback plan in case of any issues. It's also important to communicate the changes to any team members or stakeholders involved in the project.
In summary, the future of MySQL connections in PHP is moving towards more modern and secure methods, such as PDO and MySQLi. Developers need to start preparing for the changes now by updating their code to use these methods. Having a plan in place, staying informed about future developments in PHP and MySQL, and being prepared for a continuous process of updating and adapting will help to make the transition as smoothly as possible.
Questions and Answers
Q: What is the mysql_connect() function?
A: mysql_connect() is a function used to establish a connection to a MySQL database using the MySQL extension, which is now deprecated. This function takes three parameters: the server hostname, the username, and the password for the database.
Q: Why is the mysql extension deprecated?
A: The mysql extension has been deprecated as of PHP 5.5 and removed as of PHP 7.0 because it is not maintained anymore and has been superseded by the improved MySQLi and PDO_MySQL extensions. Furthermore, the mysql extension also has several security issues and not support for new features included in recent versions of MySQL.
Q: What should I use instead of mysql_connect()?
A: Instead of mysql_connect() you should use either the MySQLi extension or the PDO_MySQL extension. Both of these extensions offer improved security and support for new features included in recent versions of MySQL. MySQLi provides an object-oriented interface, while PDO_MySQL provides a data-access abstraction layer, which means that it can work with multiple databases, not just MySQL.
Q: How do I use MySQLi to connect to a database?
A: To connect to a MySQL database using MySQLi, you can use the mysqli_connect() function. The function takes four parameters: the server hostname, the username, the password, and the database name. Once connected, you can use the mysqli_query() function to execute queries on the database. Here is an example of how to connect to a database using MySQLi:
$db = mysqli_connect('hostname', 'username', 'password', 'database_name');
Q: How do I use PDO_MySQL to connect to a database?
A: To connect to a MySQL database using PDO_MySQL, you can use the PDO() constructor. The constructor takes four parameters: the DSN (data source name), the username, the password, and an array of options. Once connected, you can use the PDO::query() method to execute queries on the database. Here is an example of how to connect to a database using PDO_MySQL:
$db = new PDO('mysql:host=hostname;dbname=database_name', 'username', 'password');
Q: What is the difference between MySQLi and PDO_MySQL?
A: MySQLi and PDO_MySQL are both extensions used to connect to a MySQL database in PHP. The main difference between them is the way they handle the connection and the way they handle data. MySQLi is an object-oriented extension, whereas PDO_MySQL is a data-access abstraction layer. This means that MySQLi handles the connection and data using objects, whereas PDO_MySQL handles it using a single class. Additionally, PDO_MySQL can be used to connect to multiple databases, not just MySQL, as it uses a consistent API across different databases. MySQLi, on the other hand, is specific to MySQL.
Q: What is the benefit of using PDO_MySQL over MySQLi?
A: One of the main benefits of using PDO_MySQL over MySQLi is that it can be used to connect to multiple databases, not just MySQL. This means that the same code can be used to connect to different databases, such as Oracle, SQLite, etc. Additionally, PDO_MySQL uses a consistent API across different databases, making it easier to switch between databases if needed. Furthermore, PDO_MySQL also provides better protection against SQL injection attacks by using prepared statements and bound parameters, which MySQLi do not have.
Q: How can I migrate my existing code from using mysql_connect() to using MySQLi or PDO_MySQL?
A: Migrating your existing code from using mysql_connect() to using MySQLi or PDO_MySQL can be done in several steps:
- First, you should replace the mysql_connect() function with the appropriate function for the extension you are using (mysqli_connect() for MySQLi, or PDO() constructor for PDO_MySQL)
- Next, you should replace the mysql_query() function with the appropriate function for the extension you are using (mysqli_query() for MySQLi, or PDO::query() for PDO_MySQL)
- You should also update any mysql_* functions with their corresponding MySQLi or PDO_MySQL function
- Finally, you should test your code to make sure that everything is working correctly and that there are no errors.
It's important to note that the process of migrating can vary depending on the complexity of your code and the number of mysql_* functions used.
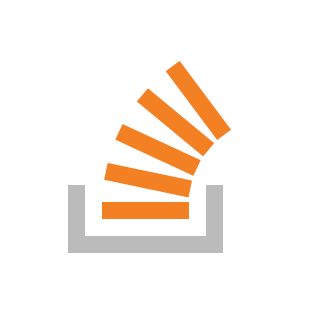