To fix an error in a query to count the number of invoices, you need to first identify the error by checking the error message.
Here's an example of a query in SQL to count the number of invoices:
SELECT COUNT(*) FROM invoices;
This query returns the total number of invoices in the invoices
table. The COUNT
function counts the number of rows in the table and *
means to count all columns.
If you want to get more details about the invoices, such as the date and amount, you can modify the query as follows:
SELECT invoice_date, SUM(invoice_amount) FROM invoices GROUP BY invoice_date;
This query returns the date and sum of invoice amount for each date in the invoices
table. The SUM
function adds up the values in the invoice_amount
column and GROUP BY
clause groups the results by the invoice_date
.
To continue, you can also add a HAVING
clause to filter the results based on a condition:
SELECT invoice_date, SUM(invoice_amount) FROM invoices
GROUP BY invoice_date
HAVING SUM(invoice_amount) > 1000;
This query returns the date and sum of invoice amount for each date in the invoices
table where the sum of invoice_amount
is greater than 1000. The HAVING
clause is used to filter the results based on a condition after the GROUP BY
clause has been applied.
It's important to note that the specific syntax of the query may vary depending on the database management system (DBMS) you are using. However, the basic principles remain the same.
You can also use conditional aggregation to get more details about the invoices:
SELECT invoice_date,
SUM(CASE WHEN invoice_status = 'Paid' THEN invoice_amount ELSE 0 END) AS Paid,
SUM(CASE WHEN invoice_status = 'Unpaid' THEN invoice_amount ELSE 0 END) AS Unpaid,
SUM(invoice_amount) AS Total
FROM invoices
GROUP BY invoice_date;
This query returns the date, sum of invoice_amount
for invoices with status 'Paid', sum of invoice_amount
for invoices with status 'Unpaid', and the total sum of invoice_amount
for each date in the invoices
table. The CASE
expression is used to conditionally sum the invoice_amount
based on the value in the invoice_status
column.
With this query, you can easily see the total amount of paid and unpaid invoices for each date, and the overall total for each date.
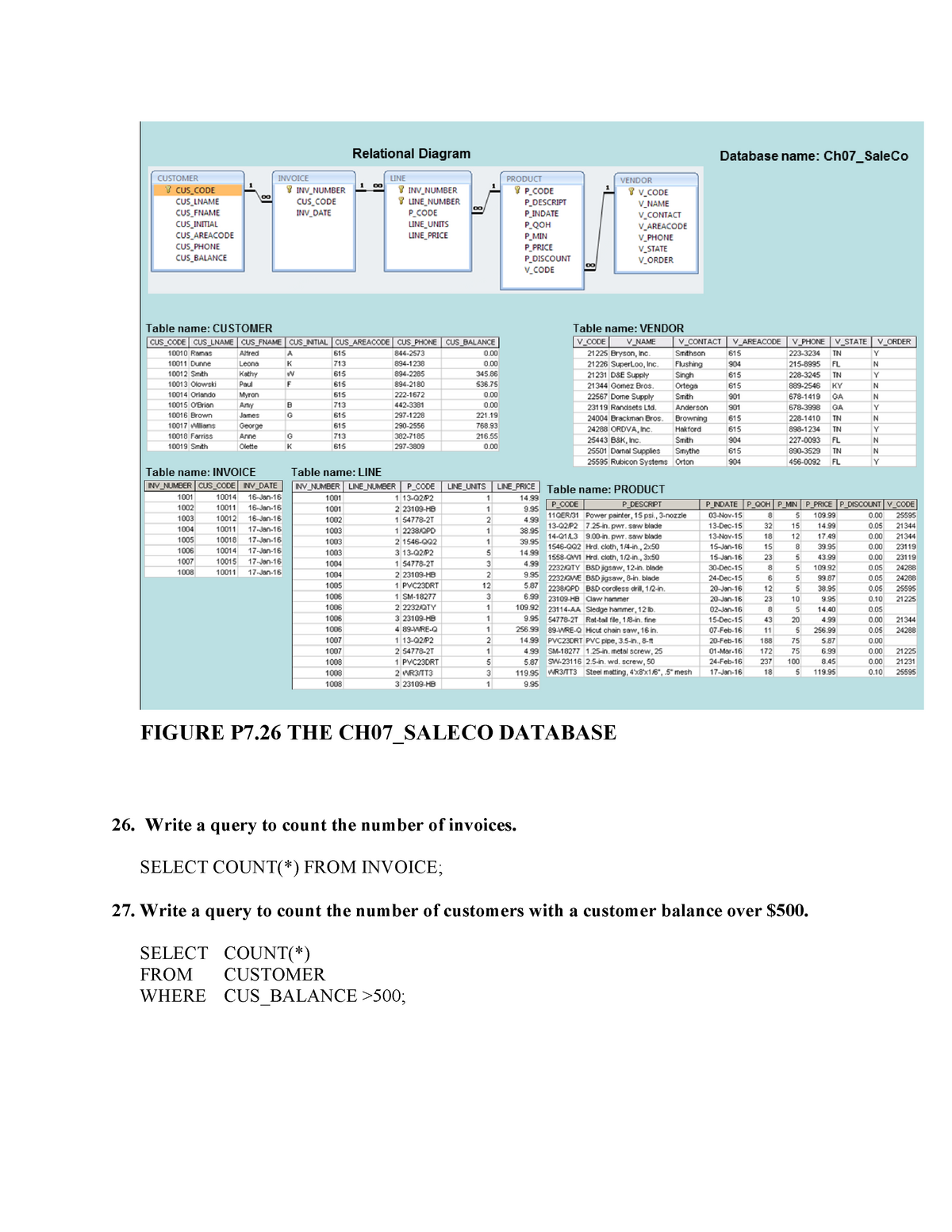
Frequently Asked Questions About The Error
What does the error message "column not found" mean?
This error message occurs when the column name specified in the query doesn't exist in the table. Check the spelling of the column name and ensure that it exists in the table.
What does the error message "incorrect syntax near" mean?
This error message occurs when there is an error in the syntax of the query. Check the query for any typos or missing elements and make sure that it follows the correct syntax for the DBMS you are using.
How can I troubleshoot a query that is taking too long to execute?
This can be due to a number of factors, such as a large number of records in the table, lack of proper indexing, or inefficient query design. You can try optimizing the query by adding indexes, reducing the amount of data being returned, or re-designing the query to make it more efficient.
What does the error message "subquery returned more than 1 row" mean?
This error message occurs when a subquery in the query is expected to return a single row, but it returns multiple rows. To fix this error, you may need to modify the subquery to ensure that it returns a single row, or use a different type of join to combine the data.
How can I prevent the error "division by zero"?
This error occurs when you try to divide a number by zero, which is undefined in mathematics. To prevent this error, you can add a conditional statement to check for division by zero and return a different result in that case.